Client-Server Data Exchange with TCP
Intro
Networking:
- Networking is a concept of communication between two entities/programms across the network:
- client to client,
- client to server,
- client to itself. - Where client is the end device usually interfacing with a human.
- Server on the other hand is a device providing a service for a client.
TCP:
- Reliable part within the protocol ensures if the data is lost on its course through the network, the prtocol will reorganize the data to be resent.
- It also checks if the data is courrpted and arrives in the ordered manner.
- However, the checking slows down the protocol.
- Applied everywhere when we need to be 100% sure the data is being completely delivered: Web Browsers, Emails, FTP...
Features
App includes following features:
Demo
Client - Server Model:
- Server can run constantly and can be available for clients to connect to at any time to receive the information clients require.
- Example: web browser as a client conncts Google as a server.
- Port number - slots for network card. Ports reroute the incoming data to appropriate program.
Sockets:
- Programming concept for establishing connection in bidirectional manner from program to program across the network.
- Once they are connected, we can use them to send data and receive data.
- One socket: server.py, another socket: client.py.
- Python sockets easily handle the implementation of the common transport protocols: TCP (Transmission Control Protocol) and UDP (User Datagram Protocol).
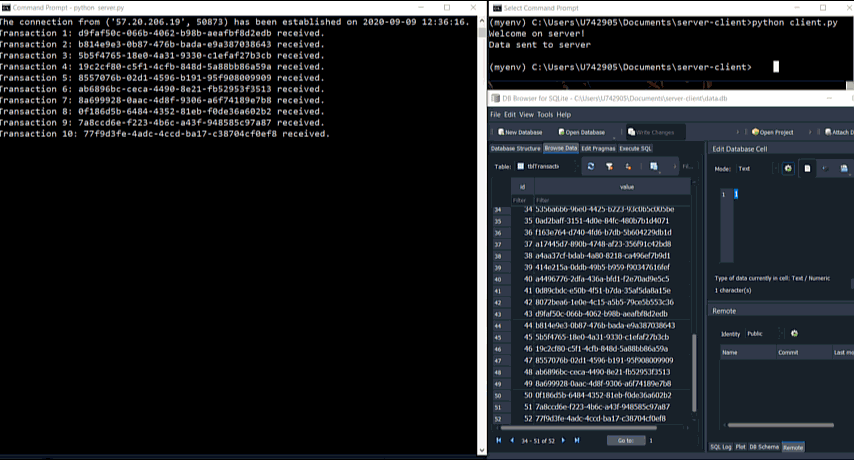
Cases study:
- Client.py connects to Server which is contantly listening for a connecton.
- Server is run from server.py.
- Client.py, once connected to Server, generates 10 random 'transactions'.
- Server confirms reception of 'transactions' batch.
- Server saves received data into db and continue to listening afterwards.
Setup
SQLite database setup:
- Creating a file in project folder: db_name.db.
- Importing sqlite3 in Python script:
import sqlite3
- Conneting to db:
conn = sqlite3.connect('db_name.db')
c = conn.cursor()
- Creating table:
c.execute('CREATE TABLE tblTransactions(id INTEGER PRIMARY KEY AUTOINCREMENT, value TEXT)')
- Inserting values:
c.execute("INSERT INTO tblTransactions VALUES(NULL, '" + row + "')")
conn.commit()
- Closing db:
c.close()
conn.close()